Update app.py
Browse files
app.py
CHANGED
@@ -5,15 +5,13 @@ from threading import Event
|
|
5 |
from typing import Optional
|
6 |
|
7 |
import discord
|
8 |
-
|
9 |
import gradio as gr
|
10 |
from discord import Permissions
|
11 |
from discord.ext import commands
|
12 |
from discord.utils import oauth_url
|
|
|
13 |
import gradio_client as grc
|
14 |
from gradio_client.utils import QueueError
|
15 |
-
from discord_slash import SlashCommand, SlashContext
|
16 |
-
from discord_slash.model import OptionData, ChoiceData
|
17 |
|
18 |
event = Event()
|
19 |
|
@@ -23,9 +21,9 @@ async def wait(job):
|
|
23 |
while not job.done():
|
24 |
await asyncio.sleep(0.2)
|
25 |
|
26 |
-
|
27 |
def get_client(session: Optional[str] = None) -> grc.Client:
|
28 |
-
client = grc.Client("https://cryptoscoutv1-discord-chatbot-
|
29 |
if session:
|
30 |
client.session_hash = session
|
31 |
return client
|
@@ -56,17 +54,22 @@ async def on_ready():
|
|
56 |
url = oauth_url(bot.user.id, permissions=permissions)
|
57 |
print(f"Add this bot to your server by clicking this link: {url}")
|
58 |
|
|
|
|
|
59 |
|
|
|
60 |
|
61 |
-
|
|
|
|
|
|
|
62 |
try:
|
63 |
-
# Acknowledge the command
|
64 |
-
await ctx.send(f"Processing
|
65 |
|
66 |
-
# Make sure this block is properly indented
|
67 |
loop = asyncio.get_running_loop()
|
68 |
client = await loop.run_in_executor(None, get_client, None)
|
69 |
-
job = client.submit(
|
70 |
await wait(job)
|
71 |
|
72 |
try:
|
@@ -78,28 +81,6 @@ async def crypto_portfolio(ctx, selection):
|
|
78 |
except Exception as e:
|
79 |
print(f"{e}")
|
80 |
|
81 |
-
# Define the options for the command
|
82 |
-
crypto_portfolio_options = [
|
83 |
-
OptionData(
|
84 |
-
name="selection",
|
85 |
-
description="Select an option",
|
86 |
-
required=True,
|
87 |
-
choices=[
|
88 |
-
ChoiceData(name="Find the top performing cryptocurrencies of the day.", value="daily"),
|
89 |
-
ChoiceData(name="Find the top performing cryptocurrencies of the week.", value="weekly"),
|
90 |
-
ChoiceData(name="Find the top performing cryptocurrencies of the month.", value="monthly")
|
91 |
-
]
|
92 |
-
)
|
93 |
-
]
|
94 |
-
|
95 |
-
# Register your command with these options using your bot's command registration system
|
96 |
-
# Example (this will vary based on how your bot is set up):
|
97 |
-
@slash.slash(name="cryptoportfolio", description="View top performing cryptocurrencies", options=crypto_portfolio_options)
|
98 |
-
async def crypto_portfolio_command(ctx, *args, **kwargs):
|
99 |
-
await crypto_portfolio(ctx, **kwargs)
|
100 |
-
|
101 |
-
|
102 |
-
|
103 |
@bot.hybrid_command(name="cshelp", description="Get information on how to use this bot.")
|
104 |
async def help_command(ctx):
|
105 |
help_message = """
|
@@ -110,6 +91,18 @@ async def help_command(ctx):
|
|
110 |
"""
|
111 |
await ctx.send(help_message)
|
112 |
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
113 |
@bot.event
|
114 |
async def on_message(message):
|
115 |
if message.author.bot:
|
@@ -125,56 +118,4 @@ def run_bot():
|
|
125 |
else:
|
126 |
bot.run(DISCORD_TOKEN)
|
127 |
|
128 |
-
threading.Thread(target=run_bot).start()
|
129 |
-
|
130 |
-
event.wait()
|
131 |
-
event.wait()
|
132 |
-
if not DISCORD_TOKEN:
|
133 |
-
welcome_message = """
|
134 |
-
## You have not specified a DISCORD_TOKEN, which means you have not created a bot account. Please follow these steps:
|
135 |
-
### 1. Go to https://discord.com/developers/applications and click 'New Application'
|
136 |
-
|
137 |
-
### 2. Give your bot a name 🤖
|
138 |
-
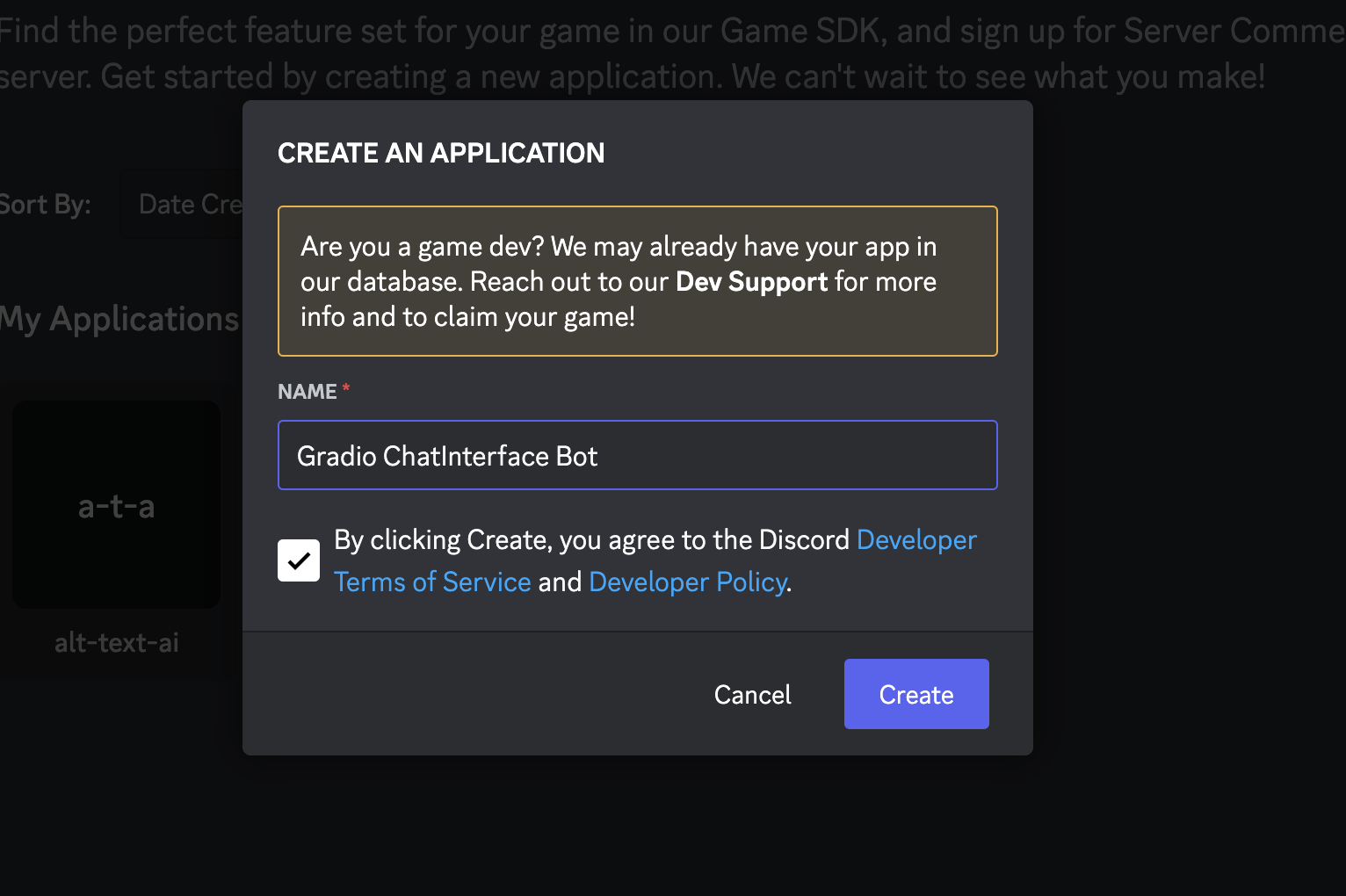
|
139 |
-
|
140 |
-
## 3. In Settings > Bot, click the 'Reset Token' button to get a new token. Write it down and keep it safe 🔐
|
141 |
-
|
142 |
-
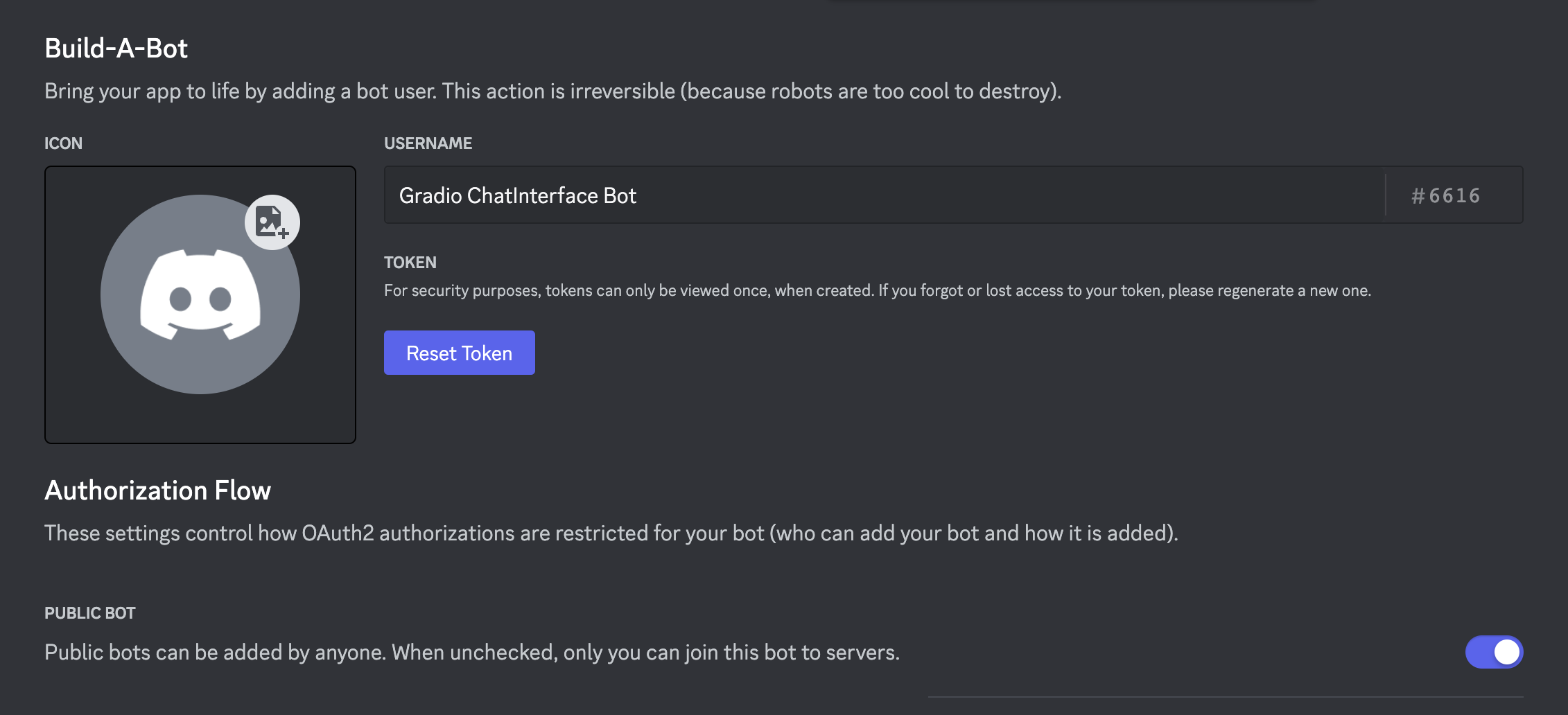
|
143 |
-
|
144 |
-
## 4. Optionally make the bot public if you want anyone to be able to add it to their servers
|
145 |
-
|
146 |
-
## 5. Scroll down and enable 'Message Content Intent' under 'Priviledged Gateway Intents'
|
147 |
-
|
148 |
-
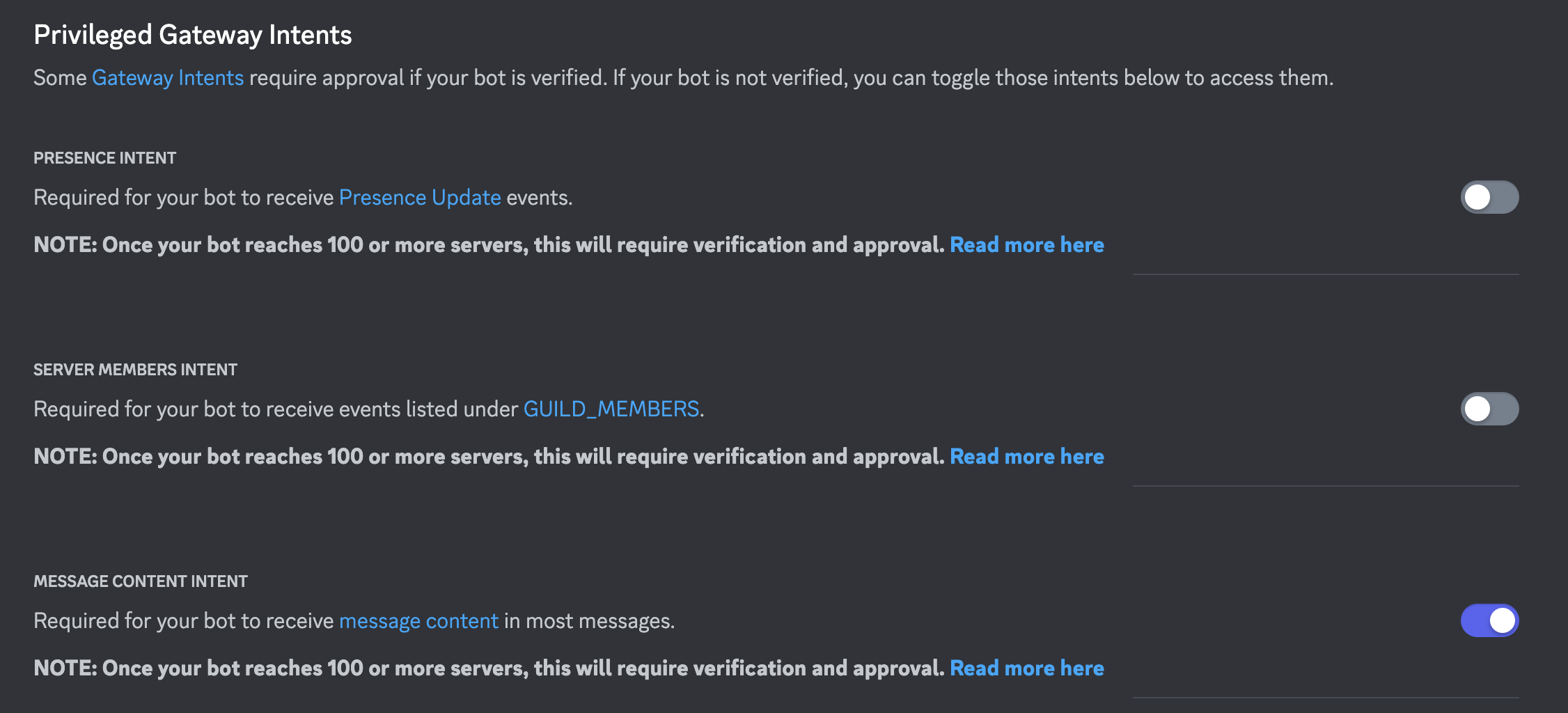
|
149 |
-
## 6. Save your changes!
|
150 |
-
## 7. The token from step 3 is the DISCORD_TOKEN. Rerun the deploy_discord command, e.g client.deploy_discord(discord_bot_token=DISCORD_TOKEN, ...), or add the token as a space secret manually.
|
151 |
-
"""
|
152 |
-
else:
|
153 |
-
permissions = Permissions(326417525824)
|
154 |
-
url = oauth_url(bot.user.id, permissions=permissions)
|
155 |
-
welcome_message = f"""
|
156 |
-
## Add this bot to your server by clicking this link:
|
157 |
-
|
158 |
-
{url}
|
159 |
-
## How to use it?
|
160 |
-
The bot can be triggered via `!echo` followed by your text prompt.
|
161 |
-
## Enabling slash commands
|
162 |
-
If you are the owner of this bot, call the `!sync` command from your discord server.
|
163 |
-
This will allow anyone in your server to call the bot via `/echo`.
|
164 |
-
This is known as a slash command and is a nicer experience than calling the bot via `!echo`.
|
165 |
-
|
166 |
-
After calling `!sync`, it may take a few minutes for `/echo` to be recognized as a valid command
|
167 |
-
in your server.
|
168 |
-
⚠️ Note ⚠️: It is best to create a separate bot per command if you intend to use slash commands. Also make sure
|
169 |
-
none of your bots have matching command names.
|
170 |
-
"""
|
171 |
-
|
172 |
-
|
173 |
-
with gr.Blocks() as demo:
|
174 |
-
gr.Markdown(
|
175 |
-
f"""
|
176 |
-
# Discord bot of CryptoSearch Portfolio Managment - Discord_CS_PM_Chatbot
|
177 |
-
{welcome_message}
|
178 |
-
"""
|
179 |
-
)
|
180 |
-
demo.launch()
|
|
|
5 |
from typing import Optional
|
6 |
|
7 |
import discord
|
|
|
8 |
import gradio as gr
|
9 |
from discord import Permissions
|
10 |
from discord.ext import commands
|
11 |
from discord.utils import oauth_url
|
12 |
+
|
13 |
import gradio_client as grc
|
14 |
from gradio_client.utils import QueueError
|
|
|
|
|
15 |
|
16 |
event = Event()
|
17 |
|
|
|
21 |
while not job.done():
|
22 |
await asyncio.sleep(0.2)
|
23 |
|
24 |
+
## GRADIO UI API LINK ##
|
25 |
def get_client(session: Optional[str] = None) -> grc.Client:
|
26 |
+
client = grc.Client("https://cryptoscoutv1-discord-chatbot-csv2.hf.space", hf_token=os.getenv("HF_TOKEN"))
|
27 |
if session:
|
28 |
client.session_hash = session
|
29 |
return client
|
|
|
54 |
url = oauth_url(bot.user.id, permissions=permissions)
|
55 |
print(f"Add this bot to your server by clicking this link: {url}")
|
56 |
|
57 |
+
thread_to_client = {}
|
58 |
+
thread_to_user = {}
|
59 |
|
60 |
+
## /echo command to return the llm repsonse ##
|
61 |
|
62 |
+
@bot.hybrid_command(name="cryptosearch", description="Enter some text to chat with the bot! Like this: /cryptosearch Hello, how are you?")
|
63 |
+
async def chat(ctx, prompt: str):
|
64 |
+
if ctx.author.id == bot.user.id:
|
65 |
+
return
|
66 |
try:
|
67 |
+
# Acknowledge the command immediately - This is the discord message after the command / is called ##
|
68 |
+
await ctx.send(f"Processing: {prompt}")
|
69 |
|
|
|
70 |
loop = asyncio.get_running_loop()
|
71 |
client = await loop.run_in_executor(None, get_client, None)
|
72 |
+
job = client.submit(prompt, api_name="/predict") ## /predict can be used for gradio interface ##
|
73 |
await wait(job)
|
74 |
|
75 |
try:
|
|
|
81 |
except Exception as e:
|
82 |
print(f"{e}")
|
83 |
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
84 |
@bot.hybrid_command(name="cshelp", description="Get information on how to use this bot.")
|
85 |
async def help_command(ctx):
|
86 |
help_message = """
|
|
|
91 |
"""
|
92 |
await ctx.send(help_message)
|
93 |
|
94 |
+
@bot.hybrid_command(name="sentences", description="Choose a predefined sentence.")
|
95 |
+
async def sentences_command(ctx):
|
96 |
+
sentences_list = [
|
97 |
+
("sentence1", "value1"),
|
98 |
+
("sentence2", "value2"),
|
99 |
+
("sentence3", "value3"),
|
100 |
+
# Add more sentences and values as needed
|
101 |
+
]
|
102 |
+
|
103 |
+
for sentence, value in sentences_list:
|
104 |
+
await ctx.send(f"{sentence} - {value}")
|
105 |
+
|
106 |
@bot.event
|
107 |
async def on_message(message):
|
108 |
if message.author.bot:
|
|
|
118 |
else:
|
119 |
bot.run(DISCORD_TOKEN)
|
120 |
|
121 |
+
threading.Thread(target=run_bot).start()
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|