Create app.py
Browse files
app.py
ADDED
@@ -0,0 +1,162 @@
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
1 |
+
import asyncio
|
2 |
+
import os
|
3 |
+
import threading
|
4 |
+
from threading import Event
|
5 |
+
from typing import Optional
|
6 |
+
|
7 |
+
import discord
|
8 |
+
import gradio as gr
|
9 |
+
from discord import Permissions
|
10 |
+
from discord.ext import commands
|
11 |
+
from discord.utils import oauth_url
|
12 |
+
|
13 |
+
import gradio_client as grc
|
14 |
+
from gradio_client.utils import QueueError
|
15 |
+
|
16 |
+
event = Event()
|
17 |
+
|
18 |
+
DISCORD_TOKEN = os.getenv("DISCORD_TOKEN")
|
19 |
+
|
20 |
+
async def wait(job):
|
21 |
+
while not job.done():
|
22 |
+
await asyncio.sleep(0.2)
|
23 |
+
|
24 |
+
#https://cryptoscoutv1-discord-cs-chatbot-2.hf.space#
|
25 |
+
def get_client(session: Optional[str] = None) -> grc.Client:
|
26 |
+
client = grc.Client("https://xxxxHGGRADIOAPIURL", hf_token=os.getenv("HF_TOKEN"))
|
27 |
+
if session:
|
28 |
+
client.session_hash = session
|
29 |
+
return client
|
30 |
+
|
31 |
+
def truncate_response(response: str) -> str:
|
32 |
+
ending = "...\nTruncating response to 2000 characters due to discord api limits."
|
33 |
+
if len(response) > 2000:
|
34 |
+
return response[: 2000 - len(ending)] + ending
|
35 |
+
else:
|
36 |
+
return response
|
37 |
+
|
38 |
+
intents = discord.Intents.default()
|
39 |
+
intents.message_content = True
|
40 |
+
bot = commands.Bot(command_prefix="/", intents=intents)
|
41 |
+
|
42 |
+
|
43 |
+
|
44 |
+
## BOT COMMANDS ##
|
45 |
+
|
46 |
+
@bot.event
|
47 |
+
async def on_ready():
|
48 |
+
print(f"Logged in as {bot.user} (ID: {bot.user.id})")
|
49 |
+
synced = await bot.tree.sync()
|
50 |
+
print(f"Synced commands: {', '.join([s.name for s in synced])}.")
|
51 |
+
event.set()
|
52 |
+
print("------")
|
53 |
+
|
54 |
+
# Generate OAuth URL when the bot is ready
|
55 |
+
permissions = Permissions(326417525824)
|
56 |
+
url = oauth_url(bot.user.id, permissions=permissions)
|
57 |
+
print(f"Add this bot to your server by clicking this link: {url}")
|
58 |
+
|
59 |
+
thread_to_client = {}
|
60 |
+
thread_to_user = {}
|
61 |
+
|
62 |
+
## /echo command to return the llm repsonse ##
|
63 |
+
|
64 |
+
@bot.hybrid_command(name="echo", description="Enter some text to chat with the bot! Like this: /echo Hello, how are you?")
|
65 |
+
async def chat(ctx, prompt: str):
|
66 |
+
if ctx.author.id == bot.user.id:
|
67 |
+
return
|
68 |
+
try:
|
69 |
+
# Acknowledge the command immediately - This is the discord message after the command / is called ##
|
70 |
+
await ctx.send("Processing your request...")
|
71 |
+
|
72 |
+
loop = asyncio.get_running_loop()
|
73 |
+
client = await loop.run_in_executor(None, get_client, None)
|
74 |
+
job = client.submit(prompt, api_name="/chat") ## /predict can be used for gradio interface ##
|
75 |
+
await wait(job)
|
76 |
+
|
77 |
+
try:
|
78 |
+
job.result()
|
79 |
+
response = job.outputs()[-1]
|
80 |
+
await ctx.send(truncate_response(response)) # Send the LLM response
|
81 |
+
except QueueError:
|
82 |
+
await ctx.send("The gradio space powering this bot is really busy! Please try again later!")
|
83 |
+
except Exception as e:
|
84 |
+
print(f"{e}")
|
85 |
+
|
86 |
+
@bot.hybrid_command(name="cshelp", description="Get information on how to use this bot.")
|
87 |
+
async def help_command(ctx):
|
88 |
+
help_message = """
|
89 |
+
**How to Use This Bot**
|
90 |
+
- Use `/echo your_message` to interact with the bot.
|
91 |
+
- Your message will be processed, and you will get a response.
|
92 |
+
- If you need any assistance, contact the server admins.
|
93 |
+
"""
|
94 |
+
await ctx.send(help_message)
|
95 |
+
|
96 |
+
@bot.event
|
97 |
+
async def on_message(message):
|
98 |
+
if message.author.bot:
|
99 |
+
return
|
100 |
+
|
101 |
+
if message.content.startswith(bot.command_prefix):
|
102 |
+
await bot.process_commands(message)
|
103 |
+
|
104 |
+
def run_bot():
|
105 |
+
if not DISCORD_TOKEN:
|
106 |
+
print("DISCORD_TOKEN NOT SET")
|
107 |
+
event.set()
|
108 |
+
else:
|
109 |
+
bot.run(DISCORD_TOKEN)
|
110 |
+
|
111 |
+
threading.Thread(target=run_bot).start()
|
112 |
+
|
113 |
+
event.wait()
|
114 |
+
if not DISCORD_TOKEN:
|
115 |
+
welcome_message = """
|
116 |
+
## You have not specified a DISCORD_TOKEN, which means you have not created a bot account. Please follow these steps:
|
117 |
+
### 1. Go to https://discord.com/developers/applications and click 'New Application'
|
118 |
+
|
119 |
+
### 2. Give your bot a name 🤖
|
120 |
+
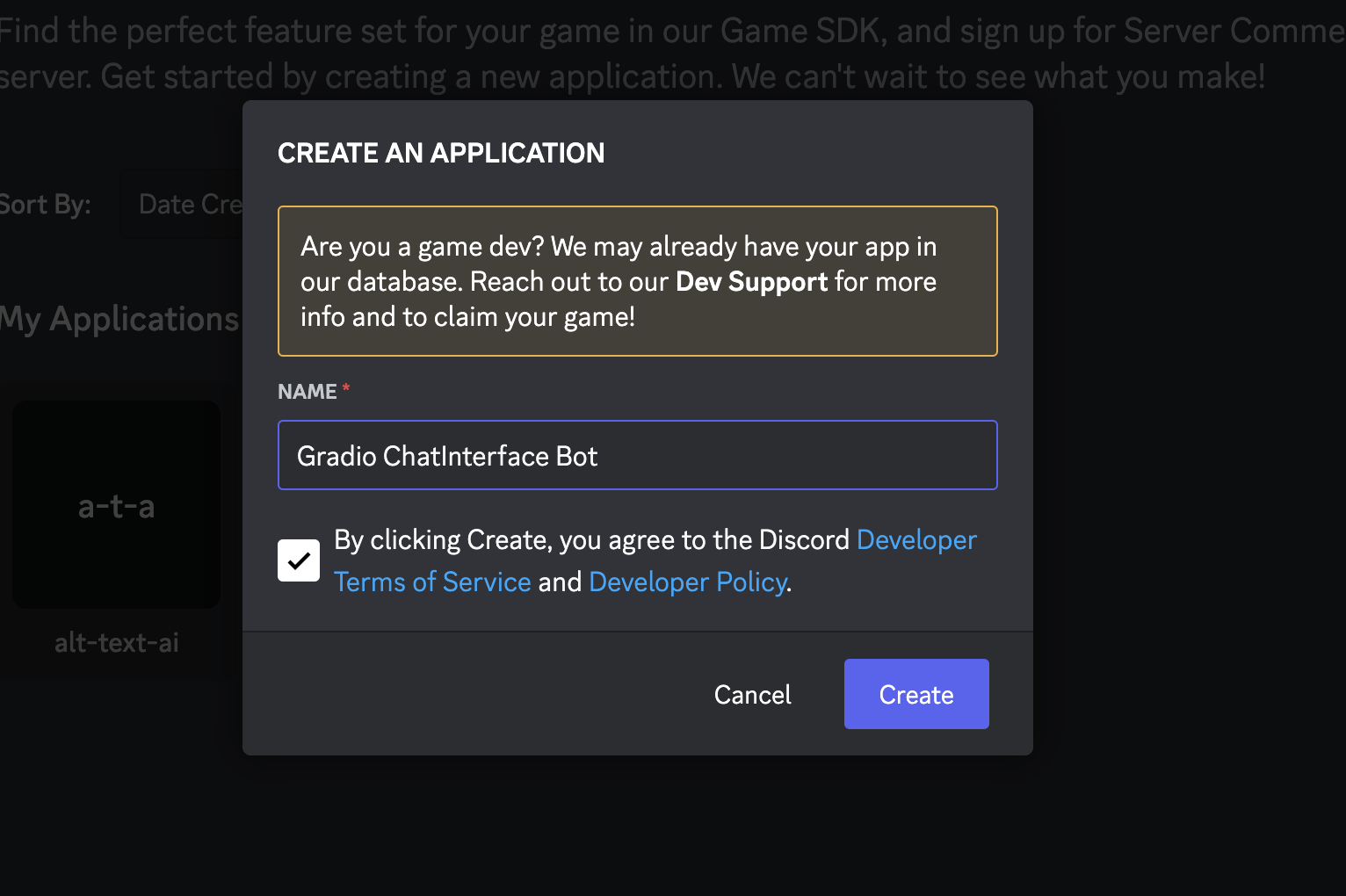
|
121 |
+
|
122 |
+
## 3. In Settings > Bot, click the 'Reset Token' button to get a new token. Write it down and keep it safe 🔐
|
123 |
+
|
124 |
+
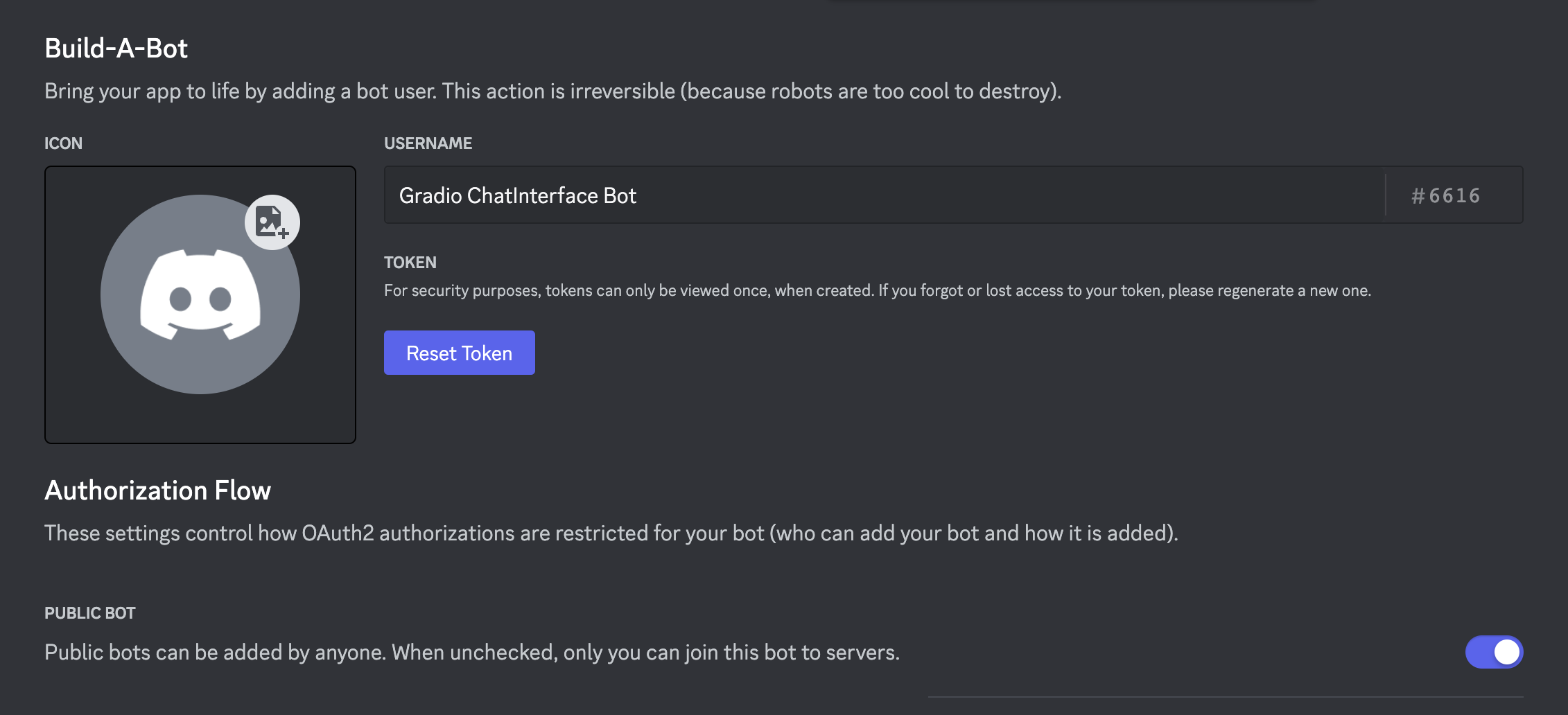
|
125 |
+
|
126 |
+
## 4. Optionally make the bot public if you want anyone to be able to add it to their servers
|
127 |
+
|
128 |
+
## 5. Scroll down and enable 'Message Content Intent' under 'Priviledged Gateway Intents'
|
129 |
+
|
130 |
+
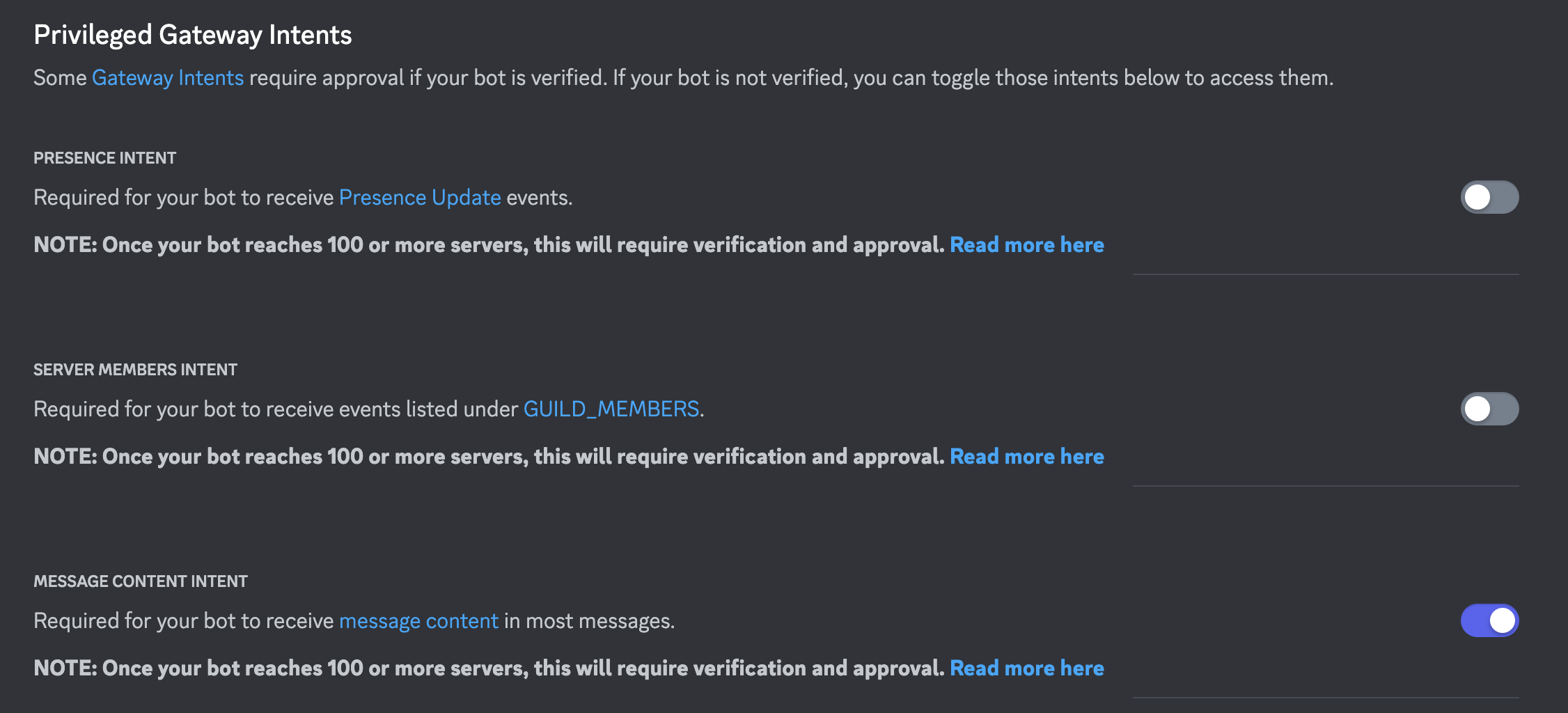
|
131 |
+
## 6. Save your changes!
|
132 |
+
## 7. The token from step 3 is the DISCORD_TOKEN. Rerun the deploy_discord command, e.g client.deploy_discord(discord_bot_token=DISCORD_TOKEN, ...), or add the token as a space secret manually.
|
133 |
+
"""
|
134 |
+
else:
|
135 |
+
permissions = Permissions(326417525824)
|
136 |
+
url = oauth_url(bot.user.id, permissions=permissions)
|
137 |
+
welcome_message = f"""
|
138 |
+
## Add this bot to your server by clicking this link:
|
139 |
+
|
140 |
+
{url}
|
141 |
+
## How to use it?
|
142 |
+
The bot can be triggered via `!echo` followed by your text prompt.
|
143 |
+
## Enabling slash commands
|
144 |
+
If you are the owner of this bot, call the `!sync` command from your discord server.
|
145 |
+
This will allow anyone in your server to call the bot via `/echo`.
|
146 |
+
This is known as a slash command and is a nicer experience than calling the bot via `!echo`.
|
147 |
+
|
148 |
+
After calling `!sync`, it may take a few minutes for `/echo` to be recognized as a valid command
|
149 |
+
in your server.
|
150 |
+
⚠️ Note ⚠️: It is best to create a separate bot per command if you intend to use slash commands. Also make sure
|
151 |
+
none of your bots have matching command names.
|
152 |
+
"""
|
153 |
+
|
154 |
+
|
155 |
+
with gr.Blocks() as demo:
|
156 |
+
gr.Markdown(
|
157 |
+
f"""
|
158 |
+
# Discord bot of TITLE HERE
|
159 |
+
{welcome_message}
|
160 |
+
"""
|
161 |
+
)
|
162 |
+
demo.launch()
|